Version: Next
DI依赖注入
1. 构造器注入
第四节说过了
2. Set注入[重点]
- 依赖:bean对象的创建依赖容器
- 注入:bean对象中的所有属性由容器来注入
2.1 环境搭建
新建一个maven模块,spring04
复杂类型
@Data@AllArgsConstructor@NoArgsConstructorpublic class Student {private String name;private Address address;private String[] books;private List<String> hobbies;private Map<String,String> cards;private Set<String> games;private String wife;private Properties info;}
beans.xml
<?xml version="1.0" encoding="UTF-8"?><beans xmlns="http://www.springframework.org/schema/beans"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://www.springframework.org/schema/beanshttps://www.springframework.org/schema/beans/spring-beans.xsd"><bean id="address" class="com.bsx.pojo.Address"><property name="address" value="我家地址"/></bean><bean id="student" class="com.bsx.pojo.Student"><!-- 普通值注入--><property name="name" value="我有名字了"/><!-- Bean注入 ref--><property name="address" ref="address"/><!-- 数组注入--><property name="books"><array><value>红楼梦</value><value>西游记</value><value>水浒传</value><value>三国演义</value></array></property><!-- List注入--><property name="hobbies"><list><value>吃</value><value>喝</value><value>睡</value></list></property><!-- Map注入--><property name="cards"><map><entry key="身份证" value="11111"/><entry key="银行卡" value="6227"/></map></property><!-- Set注入--><property name="games"><set><value>魔兽</value><value>吃鸡</value><value>LOL</value></set></property><!-- 值为null的字符串--><property name="wife"><null/></property><!-- Properties注入--><property name="info"><props><prop key="学号">20190000</prop><prop key="性别">male</prop></props></property></bean></beans>结果
Student(name=我有名字了, address=Address(address=我家地址), books=[红楼梦, 西游记, 水浒传, 三国演义], hobbies=[吃, 喝, 睡], cards={身份证=11111, 银行卡=6227}, games=[魔兽, 吃鸡, LOL], wife=null, info={学号=20190000, 性别=male})
3. 拓展方式注入
注意:使用它们需要导入相应的xml约束,去官方文档找
3.1 p命名空间
用来简化<property>
标签
新建User类
@Datapublic class User {private String name;private int age;}
userbeans.xml
<beans xmlns="http://www.springframework.org/schema/beans"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xmlns:p="http://www.springframework.org/schema/p"xsi:schemaLocation="http://www.springframework.org/schema/beanshttps://www.springframework.org/schema/beans/spring-beans.xsd"><!-- p命名空间,可以直接注入属性--><bean id="user" class="com.bsx.pojo.User" p:name="我的名字" p:age="18"/></beans>测试与结果
@Testpublic void testUser() {ApplicationContext context = new ClassPathXmlApplicationContext("userbeans.xml");User user = context.getBean("user", User.class);System.out.println(user);}User(name=我的名字, age=18)
3.2 c命名空间
对应构造器注入,简化。
userbeans.xml
<!-- 命名空间,可以简化构造器注入--><bean id="user2" class="com.bsx.pojo.User" c:name="构造方法注入名字" c:age="18"/>测试与结果
@Testpublic void testUser2() {ApplicationContext context = new ClassPathXmlApplicationContext("userbeans.xml");User user = context.getBean("user2", User.class);System.out.println(user);}User(name=构造方法注入名字, age=18)
4. bean的作用域
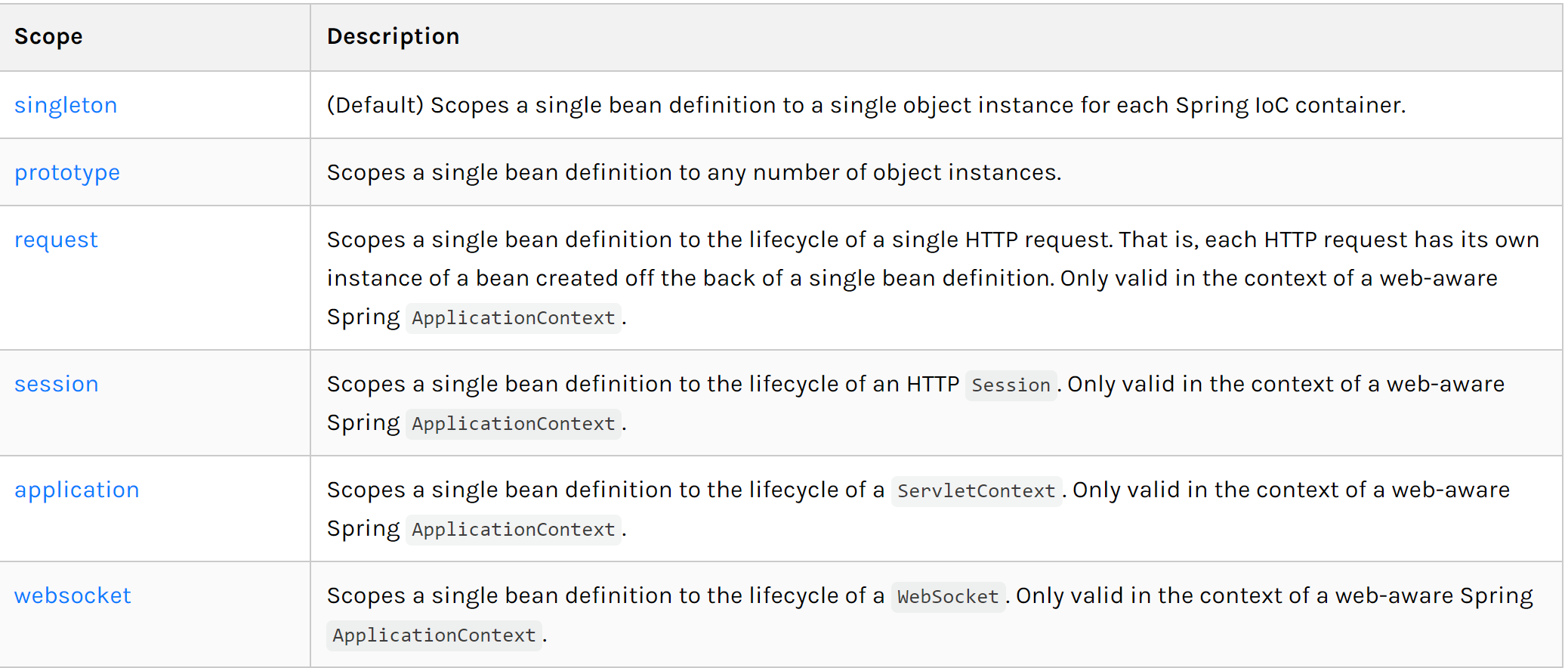
4.1 单例模式(Spring默认)
容器里的对象是单例 singleton
<bean id="user1" class="com.bsx.pojo.User" p:name="我的名字" p:age="18" scope="singleton"/>
4.2 原型模式
容器里的每一个bean都创建一个新对象 prototype
<bean id="user" class="com.bsx.pojo.User" p:name="我的名字" p:age="18" scope="prototype"/>
4.3 其他作用域
- Request:每一次HTTP请求都会产生一个新的bean,该bean尽在当前HTTP request中有效
- session:每一次HTTP请求都会产生一个新的bean,该bean只在当前HTTP的session中有效
- global-session:Spring 5已经没了
info
request、session、application、websocket只在web开发中使用