Version: Next
Ajax
- AJAX:Asynchronous JavaScript and XML——异步JavaScript和XML
- AJAX是一种无需重新加载整个网页的情况下,能够更新部分网页的技术
- AJAX不是一种新的编程语言,而是一种用于创建更好更快以及交互性更强的Web应用程序的技术
- 使用AJAX技术的网页,通过在后台服务器进行少量的数据交换,就可以实现异步局部更新
- 使用AJAX,用户可以创建接近本地桌面应用的直接、高可用、更丰富更动态的WEB用户界面
JQuery.AJAX
JQuery提供了多个与AJAX相关的方法
通过JQuery AJAX方法,我们可以使用HTTP GET和HTTP POST从远程服务器上请求文本、HTML、XML、或JSON,同时也能把这些外部数据直接载入网页被选中的元素中
JQuery AJAX的本质就是XMLHttpRequest,只是对他进行了封装,方便调用
- JQuery AJAX
参数 | 作用 |
---|---|
url | 请求地址 |
type | 请求方式、GET、POST (1.9.0之后使用method) |
headers | 请求头 |
data | 要发送的数据 |
contentType | 即将发送信息至服务器的内容编码类型,默认为application/x-www-form-urlencoded;charset=UTF-8 |
time | 设置请求超时时间(毫秒) |
beforeSend | 发送请求之前执行的函数(全局) |
complete | 完成之后执行的回调函数(全局) |
success | 成功之后执行的回调函数(全局) |
error | 失败之后执行的回调函数(全局) |
accepts | 通过请求头发送给服务器,告诉服务器当前客户端可接受的数据类型 |
dataType | 将服务器端返回的数据转换为指定类型 - "xml" - "text" - "html" - "script" - "json" - "jsonp" JSONP格式使用JSONP形式调用函数时,如 myurl?callback=? JQuery将自动替换? 为正确的函数名,以执行回调函数 |
springmvc_servlet.xml
- 开启注解支持
- 开启包扫描
- 配置默认handler
- 配置视图解析器
<?xml version="1.0" encoding="UTF-8"?><beans xmlns="http://www.springframework.org/schema/beans"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xmlns:context="http://www.springframework.org/schema/context"xmlns:mvc="http://www.springframework.org/schema/mvc"xsi:schemaLocation="http://www.springframework.org/schema/beanshttp://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/mvc https://www.springframework.org/schema/mvc/spring-mvc.xsd"><context:component-scan base-package="com.bsx.controller"/><mvc:annotation-driven/><mvc:default-servlet-handler/><bean id="internalResourceViewResolver"class="org.springframework.web.servlet.view.InternalResourceViewResolver"><property name="prefix" value="/WEB-INF/jsp/"/><property name="suffix" value=".jsp"/></bean></beans>web.xml
- 字符过滤器
- dispatcherServlet
<filter><filter-name>characterEncoding</filter-name><filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class><init-param><param-name>encoding</param-name><param-value>utf-8</param-value></init-param></filter><filter-mapping><filter-name>characterEncoding</filter-name><url-pattern>/*</url-pattern></filter-mapping><servlet><servlet-name>dispatcherServlet</servlet-name><servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class><init-param><param-name>contextConfigLocation</param-name><param-value>classpath:springmvc_servlet.xml</param-value></init-param><load-on-startup>1</load-on-startup></servlet><servlet-mapping><servlet-name>dispatcherServlet</servlet-name><url-pattern>/</url-pattern></servlet-mapping>AjaxController
@Controllerpublic class AjaxController {@RequestMapping("/a1")public void ajax1(String name, HttpServletResponse response) throws IOException {if ("admin".equals(name)) {response.getWriter().print("true");} else {response.getWriter().print("false");}}}导入JQuery js文件,可以使用在线的CDN,也可以从本地导入
在线
<script src="https://code.jquery.com/jquery-3.1.1.min.js"></script>本地
<script src="${pageContext.request.contextPath}/statics/js/jquery-3.1.1.min.js"></script>
index.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %><html><head><title>$Title$</title><script src="https://code.jquery.com/jquery-3.1.1.min.js"></script><script>function func() {$.post({url: "${pageContext.request.contextPath}/a1",data: {'name': $("#textId").val()},success: function (data, status) {alert(data);alert(status);}});}</script></head><body>用户名<input type="text" id="textId" name="textName" onblur="func()"></body></html>测试,输入admin返回true,否则返回false
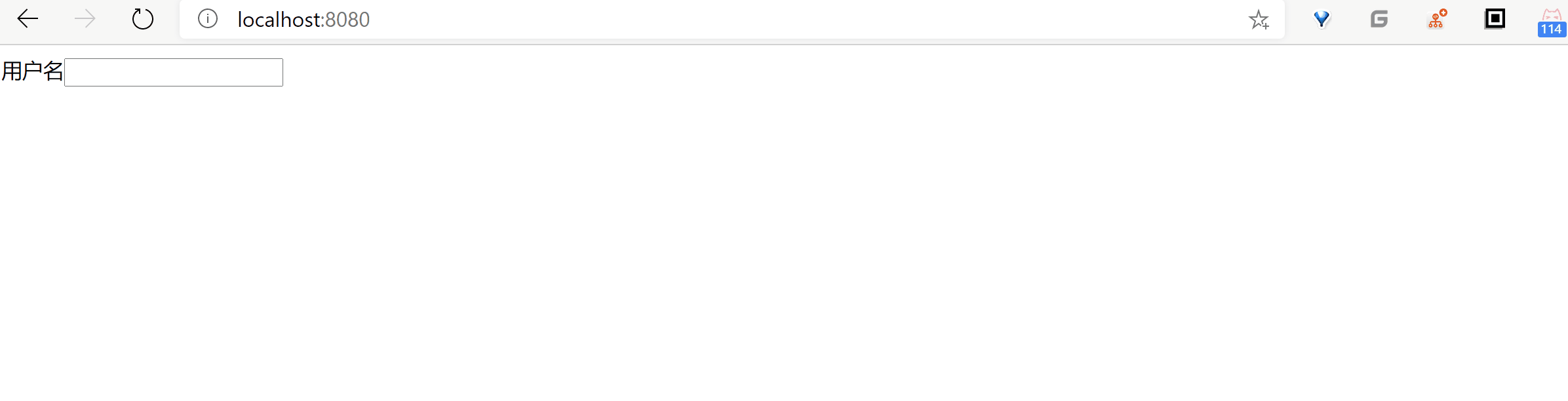
- JavaScript
- DOM
- 通过id、name、tag找到元素
- CRUD操作
- BOM
- window时间
- document
- DOM
SpringMVC实现——返回POJO对象
pojo
@Data@NoArgsConstructor@AllArgsConstructorpublic class User {private String name;private int age;private String sex;}Controller
@RequestMapping("/a2")@ResponseBodypublic List<User> a2() {ArrayList<User> userList = new ArrayList<User>();userList.add(new User("bb", 18, "男"));userList.add(new User("alice", 28, "女"));userList.add(new User("matt", 38, "男"));return userList;}直接访问
/a2
路由,可以看到后端返回的userList
Json[{"name":"bb","age":18,"sex":"男"},{"name":"alice","age":28,"sex":"女"},{"name":"matt","age":38,"sex":"男"}]test2.jsp
- 可以在
$.post
的参数列表里直接简写url,回调函数等内容,不需要写到方法体里 data
参数可省略
<%@ page contentType="text/html;charset=UTF-8" language="java" %><html><head><title>Title</title><script src="https://code.jquery.com/jquery-3.1.1.min.js"></script><script>$(function () {$("#btnId").click(function () {//$,post(url, param[可省略], success回调方法)$.post("${pageContext.request.contextPath}/a2", function (data) {var html = "";for (var i = 0; i < data.length; i++) {html += "<tr>" +"<td>" + data[i].name + "</td>" +"<td>" + data[i].age + "</td>" +"<td>" + data[i].sex + "</td>" ++"</tr>"}//往id为cotent的表格中加数据$("#content").html(html);})})})</script></head><body><input type="button" value="加载数据" id="btnId"><table><tr><td>姓名</td><td>年龄</td><td>性别</td></tr><tbody id="content"><%-- 数据在后台,需要用ajax取--%></tbody></table></body></html>- 可以在
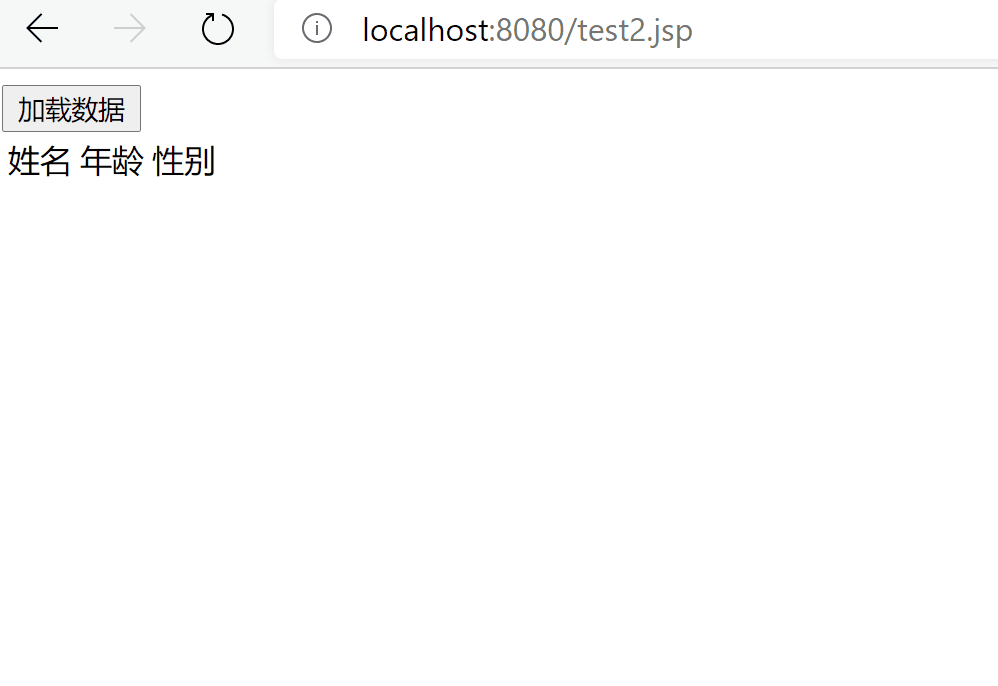